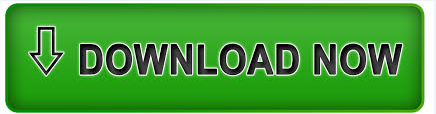
Let’s change the method printName after creating an instance: Prototypes are just objects that can be changed at runtime Adding methods on the prototypes of objects in JavaScript is an efficient way to conserve memory (as opposed to copying the methods on each object). Thus the method printName is being shared by all instances of Car. If we compare the prototypes of mazda and bmw we find that they are the same object: This object is created automatically by JavaScript when declaring a class and assigned to all the instances of the class. Where did this prototype object came from? This method was not copied when creating mazda, it is in the prototype. Mazda._proto_.hasOwnProperty('printName') // true So we can deduce that it is somewhere in the prototype chain of mazda. It is not in the object itself, but we can call it: Mazda.hasOwnProperty('printName') // false If you were to represent this object in an object literal it would look something like:
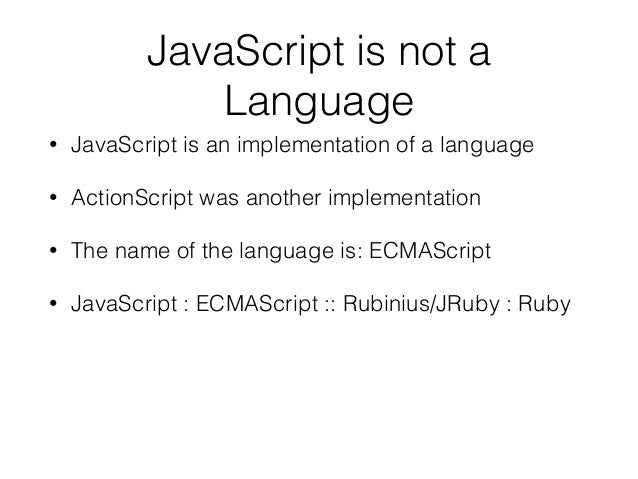
Kind and name are properties directly assigned on the new mazda object. This object gets a prototype automatically assigned to it, more on this in a bit.įirst let’s explore the attributes assigned in the constructor. To alleviate this, ES6 introduces the class syntax.Ĭlasses in ES6 don’t add any functionality to what we already have in the language, they are just a simpler syntax for building the same objects as we had before. Unfortunately Function Constructors can be quite confusing to understand (especially if you want to model inheritance).


In JavaScript we have Function Constructors, which have been the common way to build new objects until ES6.
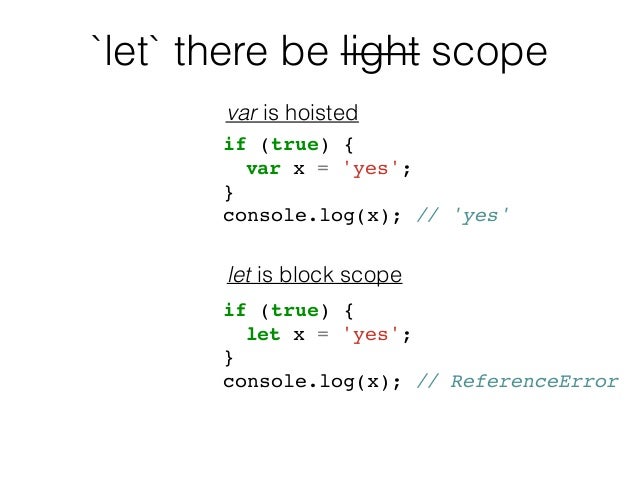
If you’d like to get a better understanding of this, please see this post. It will walk along the chain until it finds the attribute or return undefined if it can’t be found. car), it will attempt to look for that property in each object on the prototype chain (e.g. When JavaScript looks for a property that doesn’t exist in a particular object (e.g. Prototypes are simple ways to share behaviour and data between multiple objects. Let’s start with a little refresher of prototype chains in JavaScript.Įvery object in JavaScript has a special related object called the prototype. In this post I want to explain ES6 classes in the context of the JavaScript objects they produce. The class syntax just provides an alternative way to create plain old JavaScript objects. At first glance this looks like a completely new object model that follows classical languages like Java or Ruby, but behind the new syntax there is nothing new.
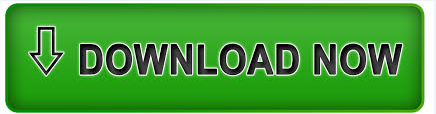